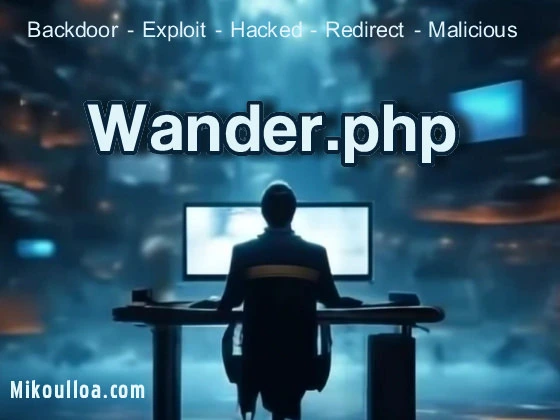
The wander.php file is a common target for cyber-attacks due to poor coding practices. This file often contains vulnerabilities that malicious actors exploit. Issues like unvalidated $_GET or $_POST input, file upload, remote code execution, and file inclusion vulnerabilities create security loopholes. Attackers can use these flaws to gain unauthorized access or control over systems.
Exploited system files, such as wander.php, allow attackers to compromise a web server. By exploiting unvalidated input, they can manipulate data requests and bypass restrictions. For instance, improper validation of $_GET or $_POST variables could expose critical files or execute harmful scripts. This oversight opens the door to remote code execution.
Unvalidated input variables pose a major security risk in PHP files. The wander.php file may accept unsanitized $_GET or $_POST requests. Hackers can exploit this vulnerability to inject malicious scripts or extract sensitive data. Developers must validate and sanitize all inputs to prevent such attacks.
Remote Code Execution (RCE) Risks
Remote Code Execution (RCE) is one of the most dangerous vulnerabilities in wander.php. When input is not validated, attackers can execute arbitrary commands on the server. For example, they might inject code through poorly sanitized form submissions. This can lead to data breaches, server hijacking, or malware installations.
Improper file upload or inclusion settings in wander.php are also critical weaknesses. Attackers exploit these vulnerabilities to upload malicious files or include unauthorized system files. For instance, file inclusion allows hackers to access sensitive directories, leading to complete server compromise. Proper file handling mechanisms can help mitigate these risks.
To secure wander.php, developers must follow security best practices. Validate all input, restrict file uploads, and enforce strict permission controls. Implementing measures like input sanitization, error handling, and server-side checks can prevent exploits. Additionally, using tools to monitor suspicious activities ensures timely threat detection.
Wander.php vulnerabilities pose a significant threat to web applications.
Exploited system files, unvalidated inputs, and file inclusion issues are serious concerns. Developers must prioritize security to mitigate remote code execution and file upload risks. By applying secure coding practices, organizations can protect their systems and prevent cyber-attacks.
Using the vulnerable script “wander.php” poses significant risks.
Firstly, it exposes your system to various critical vulnerabilities. These include exploited system files, leading to severe consequences. Furthermore, unvalidated $_GET or $_POST input creates entry points for malicious actors. Consequently, this can enable remote code execution.
Moreover, file upload and inclusion vulnerabilities compound the problem. Attackers could easily upload malicious scripts. Subsequently, these scripts could execute with the privileges of your web server. Therefore, using wander.php without thorough security measures is extremely unwise. It’s crucial to understand the risks involved.
In short, deploying wander.php without rigorous security audits is reckless. Prioritize selecting secure, well-vetted alternatives. Otherwise, you risk facing severe data breaches and system compromise. Remember, the consequences of ignoring these vulnerabilities can be devastating.
Wander.php: The Unlikely Target of Cyber Attacks
Wander.php, an innocuous-looking PHP script, has inadvertently become a hotspot for malicious activity. Security researchers have identified several vulnerabilities within this file, including unvalidated user input and potential remote code execution (RCE) risks. As a result, bots and hackers alike are eagerly crawling the web for compromised instances of wander.php to exploit.
These vulnerabilities arise primarily from the script’s lax handling of $_GET and $_POST variables, which can be manipulated by attackers to inject malicious code. Furthermore, the presence of insecure file inclusion and upload mechanisms within wander.php creates additional entry points for hackers to leveraged. Once exploited, these vulnerabilities grant attackers unrestricted access to the targeted system, paving the way for data theft, server takeover, and other nefarious activities.
The allure of wander.php to malicious actors stems from its widespread presence on many web servers, often installed as part of content management systems or distributed as free plugins. Its relative obscurity, coupled with the ease of exploitation, makes it an attractive target for script-kiddies and sophisticated hackers alike. As a result, it’s crucial for web administrators to thoroughly audit and secure their PHP scripts, paying particular attention to wander.php and similar files, to prevent these vulnerabilities from putting their systems and data at risk.
Here is an example of a vulnerable PHP script file named wander.php
that may contain a security issue such as SQL Injection—a common vulnerability often found in PHP code that interacts with databases.
Code Example: wander.php
<?php
// Vulnerable PHP Script: wander.php
// Purpose: This script demonstrates a SQL Injection vulnerability
// due to improper sanitization of user inputs.
// Database connection details (insecure example, hard-coded credentials)
$host = "localhost";
$user = "root";
$password = "password";
$db = "travelDB";
// Establishing a connection to the database
$conn = mysqli_connect($host, $user, $password, $db);
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Capture user input from the GET parameter 'location'
$location = $_GET['location'];
// SQL query without input sanitization (vulnerable to SQL Injection)
$query = "SELECT * FROM destinations WHERE city = '$location'";
// Execute the query
$result = mysqli_query($conn, $query);
// Display results
if ($result) {
while ($row = mysqli_fetch_assoc($result)) {
echo "City: " . $row['city'] . "<br>";
echo "Country: " . $row['country'] . "<br>";
echo "Description: " . $row['description'] . "<br><br>";
}
} else {
echo "Error: " . mysqli_error($conn);
}
// Close the database connection
mysqli_close($conn);
?>
Description of the Vulnerability
This script is vulnerable to SQL Injection because it takes user input ($_GET['location']
) and directly incorporates it into the SQL query without proper sanitization or parameterization.
How the Attack Works:
If a malicious user supplies a specially crafted input like this:
http://example.com/wander.php?location=' OR '1'='1
The SQL query becomes:
SELECT * FROM destinations WHERE city = '' OR '1'='1'
This query returns all rows from the destinations
table because '1'='1'
is always true. This exposes sensitive data and could escalate to further attacks if left unaddressed.
Secure Version of the Script
To fix this vulnerability, use prepared statements with parameterized queries to prevent SQL Injection. Here’s the improved version:
<?php
// Secure Version of wander.php using prepared statements
$host = "localhost";
$user = "root";
$password = "password";
$db = "travelDB";
// Database connection
$conn = mysqli_connect($host, $user, $password, $db);
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Capture user input
$location = $_GET['location'];
// Use prepared statements to prevent SQL Injection
$stmt = mysqli_prepare($conn, "SELECT * FROM destinations WHERE city = ?");
mysqli_stmt_bind_param($stmt, "s", $location);
mysqli_stmt_execute($stmt);
$result = mysqli_stmt_get_result($stmt);
// Display results
if ($result) {
while ($row = mysqli_fetch_assoc($result)) {
echo "City: " . $row['city'] . "<br>";
echo "Country: " . $row['country'] . "<br>";
echo "Description: " . $row['description'] . "<br><br>";
}
} else {
echo "No results found.";
}
// Close connections
mysqli_stmt_close($stmt);
mysqli_close($conn);
?>
- Vulnerable Code: Directly injecting user input into SQL queries leads to SQL Injection.
- Improvement: Use prepared statements with parameterized queries to sanitize user input.
- Impact of Vulnerability: Exposes data, alters queries, and compromises database security.
Always validate and sanitize user inputs to secure PHP scripts!
In the realm of web security, exploited system files pose a significant threat. These vulnerabilities, including Unvalidated $_GET or $_POST Input, Remote Code Execution, and File Upload and Inclusion Vulnerabilities, can be mitigated by utilizing the .htaccess file effectively.
Firstly, understanding the .htaccess file is crucial. This configuration file, used by Apache-based web servers, can alter server configurations on a per-directory basis. It enables server administrators to implement security measures at the directory level.
Exploited system files, such as wander.php, can be protected using .htaccess. To illustrate, consider a scenario where wander.php is vulnerable to Remote Code Execution. By adding specific directives to .htaccess, we can safeguard this file.
For instance, we can restrict access to wander.php by allowing only specific IP addresses.
This can be achieved by adding the following lines to the .htaccess file:
<Files "wander.php">
Order Deny,Allow
Deny from all
Allow from xxx.xxx.xxx.xxx
</Files>
Replace “xxx.xxx.xxx.xxx” with the IP address from which access is allowed. This restricts access to wander.php, thus preventing unauthorized Remote Code Execution.
Additionally, .htaccess can be used to block suspicious user agents.
Malicious actors often use specific user agents to exploit vulnerabilities. By blocking these user agents, we can further enhance security. This can be done by adding the following lines to .htaccess:
RewriteEngine On
RewriteCond %{HTTP_USER_AGENT} ^BadUserAgent [OR]
RewriteCond %{HTTP_USER_AGENT} ^AnotherBadUserAgent$
RewriteRule ^ - [F,L]
Here, replace “BadUserAgent” and “AnotherBadUserAgent” with the user agents you wish to block. This rule will return a 403 Forbidden error to any requests from these user agents.
Utilizing .htaccess to protect against exploited system files is an effective security measure. By implementing strategies such as IP-based access restriction and user agent blocking, we can significantly reduce the risk of Unvalidated $_GET or $_POST Input, Remote Code Execution, and File Upload and Inclusion Vulnerabilities.
Exploited system files, such as wander.php, can lead to
Remote Code Execution and Unvalidated $_GET or $_POST Input vulnerabilities. File Upload and Inclusion Vulnerabilities may also arise. To safeguard your website, let’s explore how a well-configured robots.txt file can help protect wander.php.
Understanding Robots.txt: Robots.txt is a text file that webmasters use to instruct search engine bots about indexing their website. It’s beneficial for both SEO and security. However, robots.txt is not a substitute for proper security measures, but rather an additional layer of protection.
Exploited System File: Wander.php,
being an exploited system file, can be targeted by malicious actors. Blocking such files from search engine bots using robots.txt can prevent their indexing and potential exploitation. This won’t eliminate vulnerabilities, but it reduces visibility to potential attackers.
Creating a Robots.txt File:
To create a robots.txt file, use a plain text editor. For example, to block access to wander.php, add the following lines:
User-agent: *
Disallow: /wander.php
This code blocks all bots (User-agent: *) from accessing wander.php (Disallow: /wander.php). Ensure your robots.txt file is in the root directory of your website.
Securing File Upload and Inclusion Vulnerabilities:
File Upload and Inclusion Vulnerabilities can also be mitigated by proper use of robots.txt. Block bots from accessing sensitive directories, especially those that accept uploads or include files. Remember, robust security measures should still be in place.
Conclusion: While robots.txt is not a foolproof security solution, it can add a layer of protection against exploited system files and vulnerabilities. Blocking bots from accessing wander.php and sensitive directories can reduce visibility, making your website less attractive to potential attackers.
Shielding wander.php: Securing Your Website Against Exploitable Vulnerabilities
Websites face numerous threats. Critically, vulnerabilities like exploited system files pose serious risks. Specifically, wander.php
might be susceptible to attacks. Therefore, robust security measures are essential. These include addressing common flaws like unvalidated $_GET
or $_POST
input, remote code execution, and file upload and inclusion vulnerabilities.
Consequently, implementing security headers is a crucial first step. These headers act as a protective layer. They prevent many common attacks. Furthermore, they add an extra layer of defense against malicious actors. For instance, Content Security Policy (CSP) helps prevent cross-site scripting (XSS).
Moreover, HTTP Strict Transport Security (HSTS) enforces HTTPS.
This prevents man-in-the-middle attacks. X-Frame-Options prevents clickjacking. Additionally, X-Content-Type-Options helps mitigate MIME-sniffing vulnerabilities. These combined measures significantly enhance website security.
Specifically, protecting wander.php
requires careful consideration. This file, in particular, might be a target. Therefore, implementing robust input validation routines is vital. Sanitize all user inputs received by wander.php
. This prevents injection attacks.
For example, here’s how you might implement these headers in an Apache .htaccess
file:
Header always set X-Frame-Options "SAMEORIGIN"
Header always set X-Content-Type-Options "nosniff"
Header always set Strict-Transport-Security "max-age=63072000; includeSubDomains; preload"
Header always set Content-Security-Policy "default-src 'self'; script-src 'self' 'unsafe-inline'; img-src 'self' data:; style-src 'self' 'unsafe-inline'"
Proactive security measures are indispensable. Using security headers is a fundamental aspect of web application security. They provide a critical defense against common attacks targeting vulnerabilities like those found in wander.php
. Regular security audits and updates remain essential for ongoing protection.
Top 3 security application that could help protect your server and websites.
- Wordfence Security (https://www.wordfence.com/) – Wordfence is a robust security plugin for WordPress sites that includes features to detect and prevent attacks on your PHP files, including files like wander.php. It offers real-time threat intelligence, firewall rules, malware scanning, and more to keep your site and server secure.
- ModSecurity (https://www.modsecurity.org/) – ModSecurity is an open-source web application firewall that can be installed on your Apache or Nginx web server. It can be configured to scan and filter incoming requests, including those targeting vulnerable PHP files like wander.php. ModSecurity provides a comprehensive set of rules to detect and prevent common web application attacks.
- Sucuri Security (https://sucuri.net/) – Sucuri Security is a comprehensive security solution that includes web application firewall (WAF) rules, malware scanning, and incident response services. Their WAF can be configured to protect specific files or directories, including PHP files like wander.php, by blocking malicious requests and known attack patterns.
Remember that security is an ongoing process and requires regular updates, monitoring, and hardening of your server and application configurations to stay protected against evolving threats.
To delve deeper into the security vulnerabilities of the wander.php file
start by examining the unvalidated user input mechanisms in its PHP code. Look for instances of $_GET or $_POST variables being directly incorporated into system file paths or executed as code without proper sanitization. This lack of input validation leaves the door wide open for remote code execution attacks.
Furthermore, scrutinize any file upload and inclusion functionality within wander.php. Inspect how user-submitted files are handled, stored, and processed. Potentially malicious files could be uploaded and exploited, or included through unsafe file paths, permitting an attacker to execute arbitrary code on the server.
For a comprehensive understanding of these vulnerabilities and how to effectively mitigate them, consult the following reputable sources:
- OWASP’s PHP Security Cheat Sheet (https://cheatsheetseries.owasp.org/cheatsheets/PHP_Security_Cheat_Sheet.html)
- PHP’s Official Security Guide (https://www.php.net/security/)
- SANS Institute’s PHP Security Resources (https://www.sans.org/php-security)
By meticulously investigating the wander.php code and utilizing these trusted resources, you’ll gain the knowledge necessary to identify and remediate the identified vulnerabilities, strengthening the overall security posture of your web application.