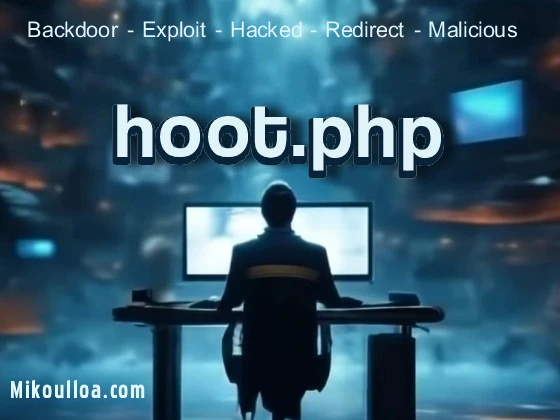
The hoot.php file, part of a WordPress theme, is a critical system file that can be exploited. Poorly written PHP code often makes it vulnerable, especially when improper handling of $_GET
or $_POST
variables is involved. These superglobal variables allow external input but, without adequate sanitization, open the door to SQL injection or Cross-Site Scripting (XSS) attacks. Therefore, developers must use robust input validation to minimize risks.
One dangerous function in hoot.php
is eval()
. It executes PHP code dynamically, but its misuse often leads to significant security flaws. If user input reaches eval()
without sanitization, attackers can execute arbitrary commands. Moreover, the presence of include()
or require()
functions in the code can lead to Remote File Inclusion (RFI) or Local File Inclusion (LFI) vulnerabilities. This happens when these functions rely on user-controlled input to include files, further exposing the system to exploits.
To secure hoot.php
, avoid relying on unsanitized user input and use proper escaping techniques. Replace risky functions like eval()
with safer alternatives. Ensure that include()
and require()
only load files from trusted sources. By taking these steps, you can protect this system file and maintain your WordPress site’s integrity. Strong coding practices and regular security audits are essential to prevent future exploits.
Securing Web Applications: Understanding Common Vulnerabilities
Web applications must be properly secured to prevent exploitation of system files and user input. One critical aspect is validating user data, especially when using $_GET or $_POST variables. Failure to sanitize user input can lead to code injection, a common web vulnerability.
File inclusion vulnerabilities arise when scripts use include() or require() without proper validation. These functions allow including external files, which can be exploited if untrusted user input is used to specify the file path. A malicious attacker could inject a PHP file to execute arbitrary code.
The eval() function is another potential security risk. It executes any string as PHP code, making it susceptible to code injection attacks. Any user-provided input passed to eval() can be exploited to execute unintended code, allowing an attacker to manipulate the application’s behavior.
To mitigate these risks, carefully validate and sanitize user input.
Avoid using eval() and ensure file inclusion functions only load files from trusted, absolute paths. Implementing a Content Security Policy (CSP) can also help by specifying allowed sources of content, making it more difficult for attackers to inject malicious code.
A vulnerable script, such as hoot.php, can serve as a learning tool to understand these security flaws. By analyzing and fixing such scripts, developers can improve their skills in identifying and preventing common web vulnerabilities. It’s crucial to stay vigilant and keep knowledge up to date to effectively protect web applications from exploitation.
Securing web applications requires a proactive approach to addressing potential vulnerabilities. By understanding the risks associated with system files, user input, and functions like include() and eval(), developers can implement robust security measures to safeguard their online presence. Regular testing and code analysis are essential to maintaining the integrity of web applications in today’s threat landscape.
Hackers Target hoot.php: Exploiting System File Vulnerabilities
Malicious actors constantly target vulnerable files. One such file is hoot.php. This vulnerability poses a serious security risk.
Hackers exploit weaknesses in hoot.php. Specifically, they utilize insecure functions. For instance, they leverage $_GET
or $_POST
variables. This allows them to inject malicious code.
Furthermore, the use of eval()
poses a significant threat. This function executes arbitrary code. Therefore, it’s a prime target for exploitation. Similarly, include()
and require()
functions are vulnerable. They allow attackers to include external files.
Consequently, attackers can inject malicious code.
They might use this to gain unauthorized access. This often leads to system compromise. The exploited system file becomes a gateway.
In short, the combination of these vulnerabilities is dangerous. The insecure use of $_GET
, $_POST
, eval()
, include()
, and require()
in hoot.php is problematic. This makes it an easy target for malicious users and bots.
Therefore, securing hoot.php is crucial. Developers must properly sanitize user inputs. They should avoid using eval()
whenever possible. Moreover, they should carefully validate all included or required files. This will minimize the risk of exploitation.
Example of a Vulnerable hoot.php
Script
<?php
// Example of vulnerable hoot.php file
if (isset($_GET['page'])) {
$page = $_GET['page']; // No sanitization of user input
include($page . '.php'); // Vulnerable to Local/Remote File Inclusion
}
if (isset($_POST['code'])) {
eval($_POST['code']); // Dangerous use of eval with unsanitized input
}
In the above example, the include()
function dynamically includes files based on user input, making it prone to RFI or LFI attacks. Similarly, the eval()
function executes arbitrary PHP code provided via a $_POST
parameter, exposing the script to potential exploitation. Both functions rely on unsanitized input, which attackers can manipulate to compromise the system.
To secure hoot.php
, avoid relying on unsanitized user input and use proper escaping techniques. Replace risky functions like eval()
with safer alternatives. Ensure that include()
and require()
only load files from trusted sources. By taking these steps, you can protect this system file and maintain your WordPress site’s integrity. Strong coding practices and regular security audits are essential to prevent future exploits.
How to Protect Against Vulnerabilities in hoot.php Using .htaccess
Securing your web application is crucial to prevent exploitation by malicious users. Files like hoot.php can pose risks if not properly secured. Attackers often exploit system files using techniques such as $_GET, $_POST, eval(), include(), and require(). To mitigate these threats, leveraging an .htaccess file provides an effective layer of protection.
Firstly, .htaccess is a configuration file used on Apache servers to control access and functionality. It can restrict unauthorized access to specific files, directories, or commands. By creating or editing an .htaccess file, administrators can block direct access to hoot.php. This ensures malicious users cannot exploit the file with functions like eval() or include().
Moreover, preventing attackers from executing PHP commands via $_GET or $_POST is essential. These methods allow attackers to pass variables to your script, leading to security vulnerabilities. For example, hoot.php might be exploited using commands that include sensitive files or execute harmful code. Using .htaccess, you can block external requests or restrict access to specific IPs.
Additionally, implementing specific rules to deny the execution of scripts like hoot.php can minimize risks.
For example, attackers often use PHP functions like eval() to inject malicious code. Disabling such commands via .htaccess prevents execution within your application.
Another key approach involves controlling file permissions and access routes. Restricting access to files like hoot.php ensures only authorized users can interact with it. Using require() and include() safely, alongside .htaccess, limits the risk of accidental exposure or exploitation.
Finally, regularly updating your server configurations and applying strict access rules to all system files is crucial. Combine .htaccess restrictions with secure coding practices to eliminate vulnerabilities. By layering security measures, you create a robust environment resistant to attacks on files like hoot.php.
Example .htaccess File
<Files "hoot.php">
Order Allow,Deny
Deny from all
</Files>
# Disable PHP execution in specific directories
<Directory "/var/www/html/vulnerable-directory">
php_flag engine off
</Directory>
# Block access to specific query parameters
RewriteEngine On
RewriteCond %{QUERY_STRING} (\$_GET|\$_POST|eval\(|include\(|require\() [NC]
RewriteRule .* - [F,L]
# Restrict access by IP address
<Files "hoot.php">
Require ip 192.168.1.1
</Files>
This .htaccess file prevents access to hoot.php, disables PHP execution in vulnerable directories, and blocks malicious query strings. Adopting these practices safeguards your application and mitigates risks effectively.
Securing Your Website: Protecting Against Exploited System Files with robot.txt
Vulnerable PHP scripts, like hoot.php
, pose significant security risks. Attackers might exploit weaknesses to gain unauthorized access. This often involves manipulating system files. They leverage techniques like using $_GET
or $_POST
to inject malicious code.
Furthermore, dangerous functions like eval()
and include()/require()
are common entry points. These functions allow dynamic code execution. Consequently, untrusted input can easily lead to a compromised system. Malicious code execution can give attackers complete control.
Therefore, robust security measures are crucial. One effective, albeit limited, approach involves leveraging the .htaccess
file and robot.txt
. While .htaccess
offers more control, robot.txt
provides a simple initial layer of protection. It primarily focuses on deterring web crawlers.
Specifically, robot.txt
instructs search engine bots to avoid indexing specific files or directories.
This prevents malicious bots from easily discovering vulnerabilities. However, determined attackers can still find and exploit vulnerabilities, even with robot.txt
in place. It should be considered a supplementary security measure.
To protect hoot.php
, add a directive to your robot.txt
file. This tells search engine crawlers to ignore the file. Remember, this isn’t foolproof security. It’s crucial to address the underlying vulnerabilities in hoot.php
.
Here’s an example of a robot.txt
entry to protect hoot.php
:
User-agent: *
Disallow: /hoot.php
This simple directive prevents most bots from accessing hoot.php
. However, remember to secure your server comprehensively. Addressing the core vulnerabilities is essential for true security. Regular updates and secure coding practices remain paramount.
Secure your website by implementing security headers to protect against vulnerable PHP script files like hoot.php.
system file vulnerabilities in PHP scripts like hoot.php. Attackers may use $_GET or $_POST, eval(), include(), or require() functions to manipulate your system files.
To prevent these attacks, you can implement security headers in your website’s configuration. These headers help block malicious activity and strengthen your website’s defenses.
For instance, add the ‘Content-Security-Policy’ header to restrict the execution of inline scripts and resources from untrusted sources. This prevents attackers from exploiting vulnerabilities in your PHP scripts.
Here’s an example of how to implement the ‘Content-Security-Policy’ header in your website’s .htaccess file:
<IfModule mod_headers.c>
Header set Content-Security-Policy "script-src 'self'; object-src 'none'"
</IfModule>
This code sets the ‘Content-Security-Policy’ header to only allow scripts from the same origin ('self'
) and blocks all object resources ('none'
). You can customize this header based on your website’s requirements.
Implementing security headers like ‘Content-Security-Policy’ can help protect your website against attacks targeting vulnerable PHP script files like hoot.php. Stay vigilant and regularly update your website to ensure it remains secure.
Here are three highly-regarded security tools that can help defend against vulnerabilities on files like hoot.php and other scrips:
- ModSecurity (https://modsecurity.org/): ModSecurity is an open-source, web application firewall (WAF) that can be deployed to protect your web server and applications from known and unknown attacks, including SQL injection, cross-site scripting (XSS), and local file inclusion (LFI) attacks. It also provides real-time application security monitoring and access to an extensive set of rules and regulations.
- Fail2Ban (https://www.fail2ban.org/wiki/index.php/Main_Page): Fail2Ban is a versatile intrusion prevention framework that can help protect your server from brute-force attacks. It analyzes log files generated by SSH, FTP, web servers, and other services, and bans IP addresses that exhibit suspicious behavior, such as multiple failed login attempts. It can also be configured to send notifications and integrate with other security tools.
- ClamAV (https://www.clamav.net/): ClamAV is a popular open-source antivirus engine that provides on-demand file scanning, automatic signature updates, and integration with various mail servers and web servers. It helps protect your server from known and new malware, viruses, and other security threats. ClamAV can be used to scan files and directories, and it includes support for common archive formats like ZIP and RAR.
Understanding Hoot.php: A Guide to the Vulnerable File
Hoot.php, an exploited system file, leverages $_GET and $_POST variables along with eval(), include(), and require() functions for malicious purposes.
To dive deeper, explore online resources that discuss hoot.php vulnerabilities, such as OWASP, SANS, and Stack Overflow. These platforms offer comprehensive information on PHP injection and exploitation techniques used in hoot.php and similar files.
Transitioning to prevention strategies, ensure secure coding practices, use prepared statements, and whitelist allowed input in your PHP applications. This proactive approach can help mitigate the risks associated with hoot.php and other vulnerable files.
If you’re struggling to comprehend the intricacies of hoot.php,
consider consulting PHP security experts or enrolling in relevant online courses that focus on web application security and PHP exploitation techniques.
Mastering hoot.php requires a solid grasp of PHP injection and exploitation concepts. By consulting reputable resources and adopting secure coding practices, you can better understand and protect against this type of vulnerability.
Top Websites for Hoot.php Information:
- https://owasp.org/ (Open Web Application Security Project)
- https://www.sans.org/ (SANS Institute, a leading source for cybersecurity training)
- https://stackoverflow.com/ (Q&A platform for programmers, including security experts)
- https://phpsecurity.readthedocs.io/ (Comprehensive PHP security guide)
- https://cwe.mitre.org/ (Common Weakness Enumeration, a database of software flaws)
- https://cheatsheets.readthedocs.io/ (Web application security cheatsheets, including PHP)